Java Mastery: Exploring the Most Popular Frameworks for Modern Development
Java’s versatility and reliability have made it a go-to choice for beginners and experienced developers. As modern applications’ complexity continues to evolve, efficient tools and methodologies are needed. This is where Java frameworks play their part. They provide developers with a structured approach to application development while leveraging Java’s power.
Java frameworks have become indispensable in software engineering, facilitating rapid development and providing pre-built modules for everyday tasks. From Spring to Hibernate, Apache Struts to Play Framework, let’s explore these tools’ features, advantages, and challenges.
Table of Content:
Significance of Frameworks in Java Development
First and foremost, let’s address a fundamental question: What is a Java framework, and why should developers consider using one?
A Java framework is a pre-built set of libraries, components, and tools designed to streamline development by providing a structured foundation for building applications. They abstract away everyday tasks such as database access, input validation, and session management, allowing developers to focus more on implementing business logic and delivering value to end-users.
Moreover, frameworks promote code reusability and modularity, facilitating the development of scalable and maintainable applications. By following a consistent structure and design, developers can collaborate more efficiently and ensure code consistency across projects.
In the context of Java development, frameworks play a crucial role in accelerating development, reducing time to market, and improving overall code quality. Whether building web applications, RESTful APIs, or enterprise systems, Java frameworks let developers tackle complex challenges with confidence and efficiency.
Spring Framework
Spring is one of the most revered and widely used frameworks in the Java ecosystem. It offers a comprehensive suite of tools and modules for building enterprise-scale applications. Born out of the need to address the complexities of Java EE development, Spring provides a lightweight and flexible alternative.
At its core, Spring is an inversion of control (IoC) container that manages the lifecycle of Java objects, commonly known as beans. By delegating the responsibility of object creation and dependency injection to the Spring container, developers can decouple their application components and achieve greater modularity and testability.
Spring’s popularity can be attributed to its emphasis on simplicity, flexibility, and extensibility. Whether building RESTful APIs, microservices, or full-fledged enterprise applications, Spring provides the tools and infrastructure needed to meet the demands of modern development.
Core Features and Modules
Spring Core Container:
- Provides the foundational Inversion of Control (IoC) container.
- Manages the lifecycle of Java objects (beans) and their dependencies.
- Facilitates loose coupling and dependency injection, enhancing modularity and testability.
Spring MVC (Model-View-Controller):
- Enables the development of web applications following the MVC architectural pattern.
- Offers robust support for handling HTTP requests, mapping URLs to controller methods, and rendering views.
- Facilitates the development of RESTful APIs and web services.
Spring Boot:
- Simplifies the process of building production-ready applications with opinionated defaults and auto-configuration.
- Eliminates the need for boilerplate code and manual configuration, enabling rapid development.
- Provides embedded servers for deploying applications as standalone executables.
Spring Data:
- Simplifies data access in Java applications by providing a consistent programming model.
- Supports various data stores, including relational databases, NoSQL databases, and cloud-based services.
- Offers repository abstraction and query methods to streamline database interactions.
Spring Security:
- Provides comprehensive authentication and authorization mechanisms for securing Java applications.
- Supports features such as user authentication, access control, and session management.
- Integrates with various authentication providers, including LDAP, OAuth, and OpenID Connect.
Spring Cloud:
- Facilitates the development of cloud-native applications by providing tools for building distributed systems.
- Offers features such as service discovery, load balancing, and distributed configuration management.
- Supports microservices architectures and provides resilience patterns for building robust applications.
Spring Integration:
- Enables the integration of disparate systems and applications using messaging patterns.
- Includes support for message channels, routers, transformers, and adapters.
- Facilitates the implementation of enterprise integration patterns (EIPs) for building scalable and reliable systems.
Spring Batch:
- Facilitates the development of batch processing applications for handling large volumes of data.
- Offers features such as job scheduling, chunk-based processing, and transaction management.
- It supports parallel processing and fault tolerance to ensure the reliability of batch jobs.
Advantages and Use Cases of Spring Framework
Spring’s popularity stems from its myriad advantages and wide range of use cases, which cater to the diverse needs of modern application development. One key advantage of Spring is its ability to simplify development by providing a cohesive and consistent programming model.
Additionally, Spring’s Aspect-Oriented Programming (AOP) support allows developers to modularize cross-cutting concerns such as logging, security, and transaction management. This promotes code reusability and maintainability by encapsulating these concerns and applying them across multiple components. Spring’s robust support for declarative transaction management simplifies the management of database transactions, ensuring data integrity in multi-tiered applications.
Moreover, Spring integrates with existing technologies and frameworks, making it an ideal choice for modernizing legacy applications. Whether integrating with Hibernate for Object-Relational Mapping (ORM) or messaging systems like Apache Kafka, Spring provides the tools and abstractions to simplify integration efforts.
Potential Limitations and Mitigation Strategies
Spring boasts extensive feature sets and modular architectures, which can result in a steep learning curve. To reduce this, developers can leverage comprehensive documentation, tutorials, and community resources alongside hands-on practice to build proficiency effectively.
Performance overhead is another aspect that warrants attention. While Java frameworks offer powerful abstractions and features, they may introduce additional processing overhead, potentially impacting application performance. To address this concern, developers can implement optimization best practices, such as minimizing unnecessary dependencies and leveraging caching mechanisms, while utilizing performance profiling tools to identify and rectify bottlenecks.
Compatibility issues can arise with framework updates, necessitating modifications to existing codebases and compatibility testing. Staying informed about framework updates and release notes is essential, enabling developers to ensure compatibility with existing applications and third-party dependencies. Thorough testing and validation post upgrades are crucial to identifying and addressing compatibility issues early in the development lifecycle.
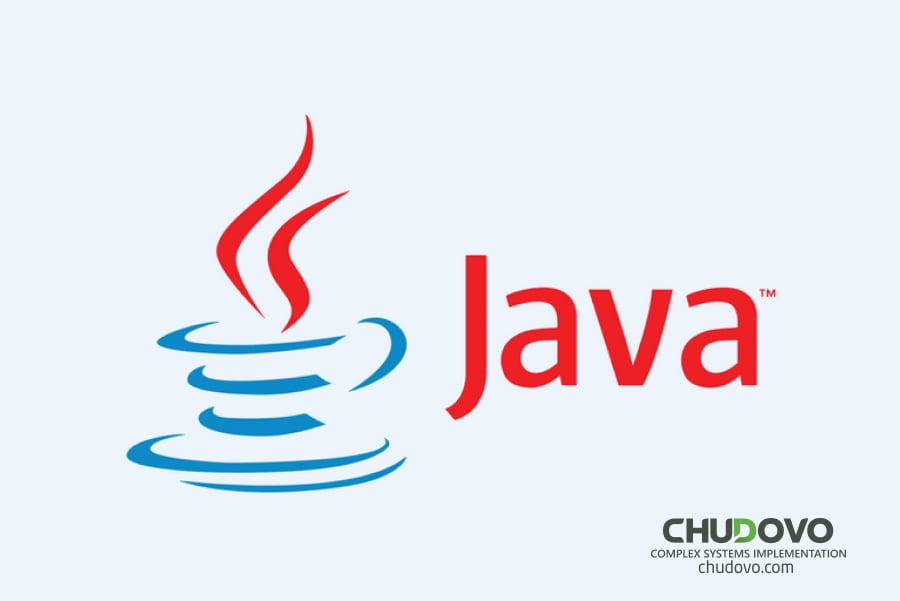
Hibernate Framework
Hibernate is one of the most influential frameworks in Java development, particularly in database interaction and Object-Relational Mapping (ORM). Emerging as a solution to the complexities associated with traditional JDBC (Java Database Connectivity) programming, Hibernate streamlines database interaction by mapping Java objects to relational database tables.
This framework provides a powerful ORM solution that bridges the gap between Java’s object-oriented programming paradigm and the relational database model. This abstraction layer enables developers to work with plain old Java objects (POJOs) without concerning themselves with low-level database operations. Hibernate handles the mapping, persistence, and retrieval of Java objects to and from the underlying database, freeing developers to focus on business logic rather than database plumbing.
Hibernate offers rich features and functionalities, including caching mechanisms, lazy loading, and support for inheritance mapping and associations. These features empower developers to build scalable and efficient database-driven applications while adhering to best practices and design patterns. No wonder Hibernate’s popularity stems from its ability to simplify database interaction and reduce development time and effort.
Core Components and Architecture of Hibernate
Session Factory:
- Responsible for creating and managing Hibernate Session instances.
- Initialized during application startup.
- Provides a factory for creating Session objects.
- Thread-safe and designed for shared usage across multiple application threads.
Session:
- Represents a single unit of work in Hibernate.
- Acts as a runtime interface between Java objects and the database.
- Encapsulates the persistence context.
- Supports CRUD operations and transaction management.
- Short-lived and created and destroyed as needed during application runtime.
Entity Mapping:
- Defines the relationship between Java objects (entities) and database tables.
- Supports various mapping strategies, including annotations and XML configuration.
- Specifies relationships such as one-to-one, one-to-many, and many-to-many associations.
Hibernate Query Language (HQL):
- A powerful query language for executing database queries against entity objects.
- Similar to SQL, it operates on entity objects rather than database tables.
- Supports features like joins, subqueries, and aggregate functions.
Transaction Management:
- An integral part of Hibernate’s architecture for ensuring data integrity and consistency.
- Supports declarative transaction management using annotations or XML configuration.
- Transactions can be managed programmatically or declaratively.
Connection Management:
- Abstracts away JDBC connection management complexities.
- Provides built-in connection pooling and management capabilities.
- Optimizes resource usage and improves application performance and scalability.
Cache Management:
- Improves application performance by reducing database round-trips and query execution time.
- It supports the first- and second-level cache (Session cache) (SessionFactory cache).
- Allows caching of entity objects and query results at different levels of granularity.
Benefits of Using Hibernate in Java Applications
Hibernate is an ORM framework for Java applications that offers numerous advantages, including streamlining database interaction and enhancing overall development efficiency. One of its primary benefits is simplifying database interaction. By abstracting the complexities of JDBC and SQL, Hibernate allows developers to interact with databases using familiar object-oriented paradigms. This abstraction significantly reduces the boilerplate code required for everyday database operations, leading to cleaner and more concise codebases.
Additionally, Hibernate provides robust Object-Relational Mapping (ORM) capabilities. It maps Java objects to relational database tables, effectively bridging the gap between the object-oriented programming paradigm and the relational database model. This mapping abstraction enables developers to work with plain old Java objects (POJOs) without needing to delve into the intricacies of low-level database operations.
Another notable benefit of Hibernate is its support for portability and database independence. By providing a layer of abstraction over database-specific SQL dialects and features, Hibernate ensures that Java applications developed with it can seamlessly transition between different relational database management systems (RDBMS) without significant code modifications.
Challenges and Considerations in Hibernate Development
For developers unfamiliar with ORM frameworks or database management, understanding Hibernate’s core concepts, such as object-relational mapping, session management, and transaction handling, can take time and effort. Investing sufficient time and effort in learning these concepts is crucial for leveraging Hibernate effectively in development projects.
Mapping complex object hierarchies to relational database tables can pose challenges in Hibernate development. Managing associations, inheritance, and polymorphic relationships requires careful design and consideration to accurately reflect the underlying database schema.
Additionally, while beneficial for performance optimization, Hibernate’s lazy loading feature can lead to the N+1 query problem if not managed properly. Developers must optimize data fetching strategies to minimize unnecessary database round-trips and improve application performance.
Concurrency and transaction management present further challenges in Hibernate development, particularly in multi-user environments. Handling transaction boundaries, isolation levels, and concurrency control mechanisms is essential to prevent data anomalies such as lost updates and dirty reads.
Apache Struts
Apache Struts is known for its reliability and adaptability in crafting scalable and easily maintainable web applications. Embracing the Model-View-Controller (MVC) architectural pattern, Struts organizes applications into distinct layers. This helps facilitate efficient business logic management, presentation, and user interaction.
At its core, Apache Struts simplifies web application development by furnishing a structured framework for constructing scalable and easy-to-maintain codebases. It abstracts away everyday web development tasks such as request handling, form validation, and navigation control, allowing developers to concentrate more on the intricacies of business requirements and application functionality.
Furthermore, Apache Struts offers robust form handling, validation, and internationalization features, making it particularly suitable for enterprise-level web applications. The framework streamlines form validation through declarative validation rules, easing the implementation of input validation and error handling.
Additionally, Apache Struts fosters reusability and modularity by supporting component-based development. Developers can encapsulate reusable components and modules within their applications, promoting code reuse and enhancing maintainability.
Key Features and Components
Model-View-Controller (MVC) Architecture:
- Apache Struts follows the MVC architectural pattern, facilitating the separation of concerns between the model (business logic), view (presentation), and controller (request handling and business logic coordination).
Action Classes:
- Controllers in Apache Struts are implemented using Action classes and are responsible for processing user requests, invoking business logic, and coordinating interactions between the model and view components.
Form Handling and Validation:
- Struts provides robust features for handling HTML form submissions, including automatic population of form fields, form validation, and error message handling.
Declarative Validation Rules:
- Developers can define validation rules declaratively using XML or annotations, simplifying the implementation of input validation and error handling.
Internationalization (i18n) Support:
- Apache Struts offers built-in support for internationalization, allowing developers to easily create multilingual web applications by externalizing message resources and supporting locale-specific content.
Custom Tag Libraries:
- Struts provides custom tag libraries for generating HTML and managing form elements, simplifying the creation of dynamic and interactive user interfaces.
Interceptors:
- Interceptors enable developers to intercept and preprocess requests and responses, allowing cross-cutting concerns such as logging, security, and performance monitoring to be easily integrated into the application.
Integration with Other Technologies:
- Apache Struts integrates with other Java technologies and frameworks, such as Hibernate for ORM, Spring for dependency injection and transaction management, and JSP for view rendering, providing developers with flexibility and choice in building web applications.
Advantages and Use Cases of Apache Struts
One of its prominent benefits lies in expediting development processes. With a structured framework and predefined architecture, Struts facilitates rapid development by offering reusable components and built-in functionalities. This feature benefits projects with tight deadlines or when swift time-to-market is paramount.
Struts’ adherence to the MVC architecture promotes modularity and scalability. By enabling developers to separate concerns, it simplifies the scaling of individual components within the application. This scalability attribute is advantageous for projects anticipating future growth and expansion, ensuring the application can accommodate increasing demands over time.
Moreover, Struts is well-equipped with features for implementing enterprise-grade security measures, such as input validation, authentication, and authorization. It provides mechanisms for safeguarding against common security vulnerabilities like cross-site scripting (XSS) and SQL injection, making it a preferred choice for developing secure web applications.
Lastly, Apache Struts benefits from a large and active community of developers, ensuring strong community support and extensive documentation. This support ecosystem empowers developers to troubleshoot issues, seek guidance, and stay updated with best practices, fostering a conducive environment for continuous learning and improvement.
Potential Limitations and Mitigation Strategies
Apache Struts has some limitations that developers can employ to mitigate. Managing the complexity of Struts configuration files, such as struts.xml, is a common challenge, particularly in large-scale projects with numerous actions and configurations.
To reduce this, developers can adhere to best practices for organizing configuration files, such as modularization, naming conventions, and thorough documentation. Additionally, employing tools and frameworks that support configuration management can streamline the configuration process and enhance maintainability.
While Struts provides a robust framework for building web applications, it may need more flexibility and customization options than other frameworks. To address this limitation, developers can leverage Struts’ extension points and plugin architecture to customize behavior and integrate with different technologies.
Lastly, mitigating security risks involves staying informed about the Apache Struts project’s latest security updates and patches. Implementing security best practices, such as input validation, output encoding, and secure authentication mechanisms, is essential for preventing common security vulnerabilities like cross-site scripting (XSS) or SQL injection.
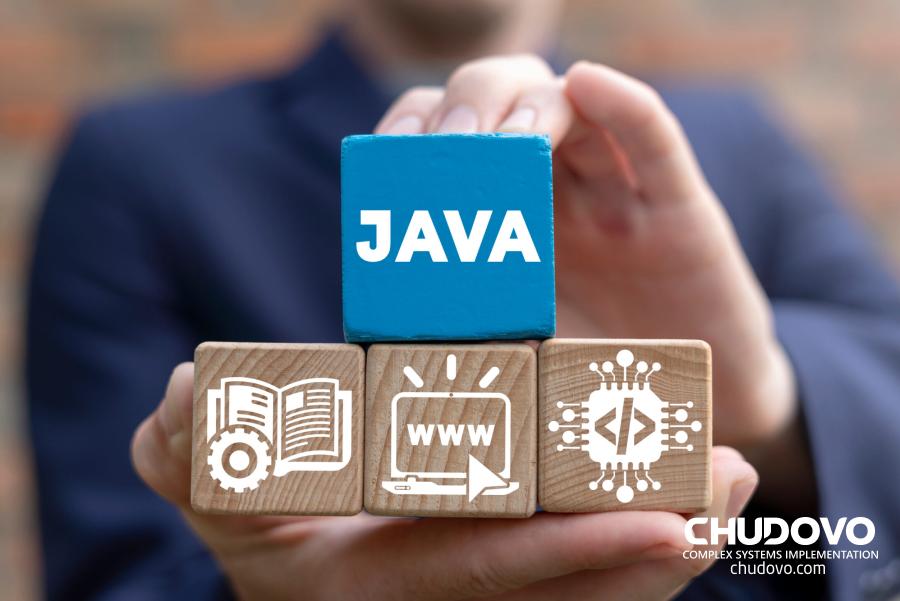
Play Framework
The Play Framework emerges as a leading Java web framework renowned for its emphasis on reactive programming principles. It offers developers a modern approach to building highly scalable and responsive web applications. Unlike traditional blocking I/O frameworks, Play leverages non-blocking I/O and asynchronous processing to handle concurrent requests.
Play Framework fosters a developer-friendly environment by embracing a convention-over-configuration philosophy. This philosophy enables rapid development and streamlined codebase maintenance. Developers can leverage Play’s intuitive APIs and built-in features to focus more on implementing business logic and less on boilerplate code, thereby enhancing productivity and code quality.
Reactive programming, a vital aspect of the Play Framework, revolves around building applications that efficiently react to events and handle asynchronous data streams. This paradigm shift from traditional imperative programming enables Play applications to handle many concurrent connections without sacrificing performance or responsiveness.
Core Components and Features
HTTP Server:
- Play Framework includes an embedded HTTP server, removing the need for external servers like Apache Tomcat or Jetty.
Routing:
- Play adopts a simple and intuitive routing mechanism, mapping HTTP requests to controller actions via a declarative syntax, simplifying request handling.
Controller Layer:
- Responsible for processing incoming HTTP requests, executing business logic, and generating responses, the controller layer promotes separation of concerns and modularity.
View Templates:
- Play supports various templating engines like Twirl and Scala templates for generating dynamic HTML content enhancing user interface development.
Forms and Data Binding:
- Play provides robust support for form handling and data binding, simplifying the creation and validation of HTML forms.
Asynchronous Programming:
- Play supports asynchronous programming, facilitating non-blocking I/O and efficiently handling concurrent requests, ideal for building responsive and scalable applications.
Dependency Injection:
- Integrated with dependency injection frameworks like Guice, Play enables efficient management of dependencies, promoting modularity and testability.
Asset Management:
- Play offers built-in support for managing static assets such as CSS, JavaScript, and images, improving application performance and load times.
Testing Support:
- Play provides comprehensive testing support, including unit, integration, and functional testing, ensuring application reliability and robustness.
Advantages of Play Framework for Modern Web Development
Play’s convention-over-configuration approach and intuitive APIs significantly boost developer productivity. Built-in features like the embedded HTTP server, robust routing mechanisms, and seamless integration with templating engines streamline common development tasks, allowing developers to focus on implementing business logic rather than dealing with boilerplate code.
The framework’s built-in support for WebSockets facilitates real-time communication between clients and servers, a critical capability for applications such as chat platforms, collaborative editing tools, and interactive dashboards. Play’s modular architecture and extensive plugin ecosystem further empower developers to extend and customize applications to meet specific requirements, whether integrating with third-party services, implementing authentication mechanisms, or optimizing performance.
Furthermore, Play Framework’s cross-platform compatibility allows developers to build and deploy applications across different operating systems, including Linux, Windows, and macOS. This flexibility empowers development teams to choose their preferred environment and deployment strategy, seamlessly integrating Play into existing workflows.
Potential Limitations and Mitigation Strategies
Despite its numerous advantages, Play Framework may present specific challenges that developers must address to ensure successful implementation. The learning curve associated with Play’s unique architecture and asynchronous programming model can be steep for developers unfamiliar with these concepts. To overcome this, comprehensive documentation, tutorials, and online resources are invaluable in providing guidance and facilitating learning.
While beneficial for scalability and responsiveness, asynchronous programming can introduce complexity for developers accustomed to synchronous paradigms. Mitigation strategies include investing in training programs focused on asynchronous programming and fostering a culture of knowledge sharing through code reviews and pair programming.
Community support and the availability of timely bug fixes and security patches may vary, particularly for older versions of Play. Developers can mitigate this risk by staying informed about the latest releases and updates, actively participating in community discussions and forums to seek assistance, and considering contributions to the Play community through bug fixes or plugin development.
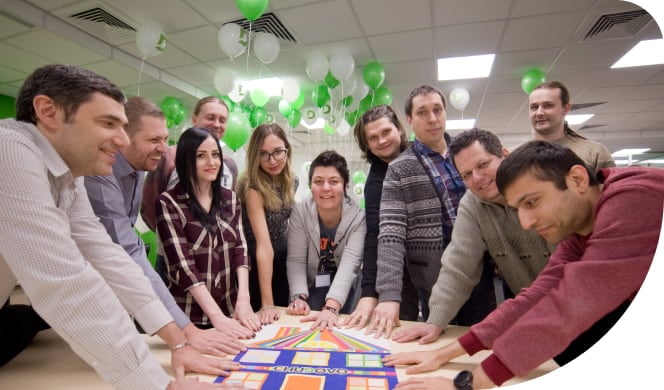
Certified engineers
Convenient rates
Fast start
Profitable conditions
Agreement with
EU company
English and German
speaking engineers
Dropwizard Framework
Dropwizard has gained significant traction in the Java ecosystem, particularly in microservices architecture—a design approach where applications are composed of small, independent services that communicate over well-defined APIs. Known for its lightweight and opinionated nature, Dropwizard framework developers a pre-configured set of libraries and tools for rapidly building web services and applications.
Dropwizard facilitates the development of RESTful APIs, a fundamental requirement in microservices architecture. It provides annotations and conventions for defining resource endpoints, request/response mappings, and error handling, streamlining the process of exposing functionalities as web services. This makes it easier for developers to design and implement scalable and interoperable microservices.
Dropwizard also offers built-in support for monitoring and operational capabilities, including health checks, metrics, and operational dashboards. These features enable developers to monitor the health and performance of microservices in real time, identify potential bottlenecks, and troubleshoot issues effectively, ensuring the reliability and resilience of microservices-based applications.
Furthermore, Dropwizard integrates seamlessly with other components and libraries in the Java ecosystem, such as Hibernate for database access, Kafka for messaging, and OAuth for authentication.
Core Components and Libraries in Dropwizard
Jetty:
- Dropwizard utilizes Jetty as its embedded HTTP server, simplifying handling HTTP requests and responses without needing external servlet containers.
Jersey:
- Jersey, the reference implementation of JAX-RS, is integrated into Dropwizard to build RESTful APIs. It provides annotations and conventions for defining resource endpoints and request/response mappings.
Jackson:
- Dropwizard incorporates Jackson, a high-performance JSON processor, for seamless JSON serialization and deserialization, enabling efficient communication between clients and web services.
Metrics:
- The framework includes Metrics, a powerful library for collecting and reporting application metrics. Metrics allow developers to monitor various performance aspects, such as response times and error rates.
Hibernate Validator:
- Dropwizard leverages Hibernate Validator for input and data validation, ensuring data integrity and consistency in web services and applications.
Guice:
- Dropwizard seamlessly integrates with Google Guice, facilitating dependency injection and promoting modular design by declaring dependencies through annotations.
Slf4j and Logback:
- Slf4j and Logback are used for logging purposes in Dropwizard. Slf4j is a logging facade, while Logback provides the logging implementation with features like configurable logging levels and appenders.
JUnit and Dropwizard Testing:
- Dropwizard includes support for unit testing and integration testing with JUnit. It also provides utilities for testing Dropwizard applications, enabling developers to validate their codebase effectively.
Advantages of Dropwizard for Building RESTful Services
Dropwizard adopts an opinionated architecture, presenting developers with a predefined and standardized set of libraries and tools. This approach alleviates decision-making burdens regarding component selection, configurations, and integrations, allowing developers to concentrate on implementing business logic and delivering features efficiently.
Integrating essential components within Dropwizard, including Jetty for HTTP, Jersey for RESTful APIs, Jackson for JSON serialization, and Metrics for monitoring, ensures compatibility and coherence across different framework elements. This integration simplifies development complexities, expediting prototyping and deployment of RESTful services.
Dropwizard is designed to optimize performance, leveraging lightweight components and efficient processing mechanisms. By employing Jetty as an embedded HTTP server and Jackson for JSON serialization, Dropwizard ensures minimal latency and high throughput, rendering it ideal for constructing high-performance RESTful services capable of efficiently handling large volumes of requests.
The Metrics library includes built-in support for monitoring and metrics, empowering developers to monitor various real-time performance metrics, such as request latency, error rates, and resource utilization. This insight enables proactive identification of bottlenecks, performance optimization, and service reliability and availability maintenance.
Configuration management in Dropwizard is simplified through YAML files, providing an intuitive and straightforward approach to configuring application settings such as server ports, database connections, and logging levels. This simplification accelerates configuration setup, enabling developers to focus on code development and feature delivery.
Challenges and Trade-offs in Dropwizard Development
One of the primary considerations is Dropwizard’s opinionated nature, which, while simplifying development by providing pre-configured components, may restrict flexibility for developers requiring customized configurations or alternative libraries. This can be limiting for projects with unique architectural requirements or those seeking greater flexibility in technology choices.
Another challenge lies in the limited flexibility inherent in Dropwizard’s integrated components and opinionated architecture. While these features contribute to the framework’s simplicity and efficiency, they may constrain developers seeking to deviate from Dropwizard’s conventions or integrate specialized functionalities. This can pose challenges for projects with specific requirements outside the framework’s standard offerings.
Managing dependencies in Dropwizard applications can also be complex, particularly when integrating third-party libraries or addressing compatibility issues between different versions of Dropwizard and its dependencies. Effective dependency management ensures compatibility and maintains application stability over time.
You might be also interested in reading about Java IDEs and text editors for efficient coding.
Conclusion
Java mastery extends beyond language proficiency. It encompasses a keen grasp of the ecosystem’s tools and frameworks. These frameworks empower developers to build robust, scalable applications from Spring to Hibernate, Dropwizard, and Apache Wicket. By embracing opportunities and navigating challenges, developers drive innovation in modern software development. As the Java ecosystem evolves, those who master it will continue shaping the future of technology.
Frequently Asked Questions
What are the most popular Java frameworks for web development?
Some of the most popular Java frameworks for web development include Spring Boot, Hibernate, Apache Struts, and Dropwizard. Each framework offers unique features and advantages, catering to different aspects of web application development. Spring Boot, for instance, provides comprehensive support for building enterprise-grade applications, while Hibernate simplifies database interaction through its object-relational mapping (ORM) capabilities. Apache Struts focuses on MVC architecture, facilitating the development of scalable and maintainable web applications, while Dropwizard excels in building RESTful services and microservices architectures.
Why should developers use Java frameworks for web application development?
Using Java frameworks for web application development offers several benefits. Firstly, frameworks provide a structured and standardized approach to development, promoting code reuse and maintainability. Additionally, frameworks often have built-in features and libraries that streamline everyday development tasks, such as database interaction, authentication, and session management.
What are the essential features to consider when selecting a Java framework?
Developers should consider various essential features when selecting a Java framework to ensure it aligns with project requirements and development goals. These features include scalability, which provides the framework can accommodate growing application demands; performance, to ensure optimal responsiveness and resource utilization; community support, to access resources, documentation, and assistance from a vibrant developer community; integration capabilities, to seamlessly integrate with other tools and technologies; and alignment with project requirements, ensuring the framework meets specific functionality, security, and compliance needs.
If you need Java frameworks developers, contact us now!